Python Programming of
Tutorialspoint is a good playground for beginners of Python programming. From basic to advance tutorials, including useful resources, it is
easy peasy lemon squeezy for beginners to learn from this site. Following contents only shows something important that I think you should notice. If you have no time to completely review all pages of the site, just "
learn by doing" from available python source codes.
Although Python is a Beginner's Language, Python supports the development of a wide range of applications from simple text processing to Web Apps.
- Python is Object-Oriented: Namespace of Object-Oriented programming style is supported so that you can regard objects as codes and data set.
- Python is Interpreted: That is Python can be integrated with shell scripts to do automatic jobs on the background of QNAP/Linux (through crontab). Of course, Python provides a better structure and support for large programs than shell scripting.
- Python is Interactive: This means that you can easily try packages with sample codes to realize how to apply the packages to do something.
Interactive Mode Programming: Write codes on the Python (
interpreter) directly.
Translate source code into some efficient intermediate representation and immediately execute. Perl, Python, MATLAB, and Ruby are examples of such kind of interpreter.
See
Install and Set environment of Python on QNAP NAS, you will find that the "python" executable file is located by a symbolic link point to "/usr/bin/python" or "/opt/bin/python". Thus, your python program should indicate the path in the first line with comment
#.
#!/usr/bin/python
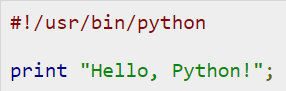 |
hello.py |
Then, execute the .py program with:
$python hello.py # Unix/Linux
Python has
no braces to indicate blocks of code for class and function definitions or flow control.
Blocks of code are denoted by line indentation, which is rigidly enforced. I.e. you should know how your text editor or IDE regard "tab" as "spaces" while saving your codes.
- Python Identifier (the name of variable, function, class, module or other object) starts with a letter A to Z or a to z or an underscore (_) followed by zero or more letters, underscores and digits (0 to 9). I.e. [A-Z|a-z|_][A-Z|a-z|_|0-9]*.
- Not allow punctuation characters such as @, $ and %.
- Case sensitive.
Naming convention for Python:
- Class names (you defined in your codes) start with an uppercase letter and all other identifiers with a lowercase letter.
- _idname is used to name private data variables.
- __idname indicates a strongly private identifier.
- __idname__ is a language-defined special name.
Notice:
- Reserved words: see http://www.tutorialspoint.com/python/python_basic_syntax.htm
- A Python Statement ends with a new line.
- Multi-Line Statements (line continuation) are concatenated with the line continuation character (\).
- Statements contained within the [], {} or () brackets do not need to use the line continuation character.
- String data are defined within quotation symbols, such as ', ", or triple quote (''' or """). String data defined within the triple quote do not need to use the line continuation character.
- Comment: #
- Multiple Statements on a Single Line are concatenated with ;.
Following codes do the same thing: open file and print the file text.
# Code 1: raw_input() # get data input
import sys; fn = raw_input("Input file name: "); file = open(fn, "r"); print file.read()
# Code 2: sys.argv[1] is the first argument, len(sys.argv)is arg counts
import sys; file = open(sys.argv[1], "r"); print file.read()
# Code 3: try-catch style programming: try-except
import systry: file = open(sys.argv[1], "r") # open existed file with "w" may empty the fileexcept IOError: print "There was an error reading to", fn sys.exit()print file.read()
 |
Code 1: raw_input(), file open() |
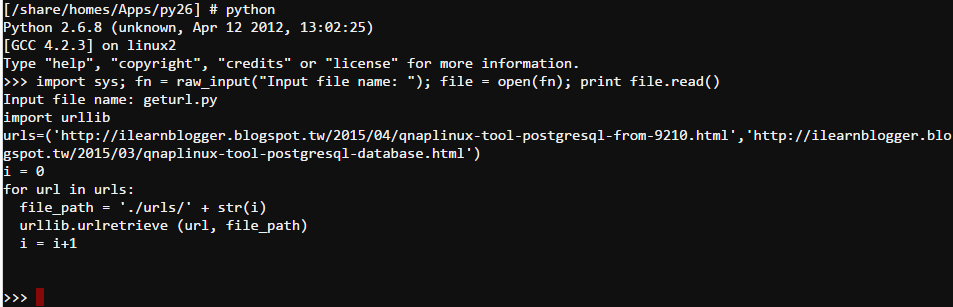 |
Run Code 1 in the interpreter's interactive mode |
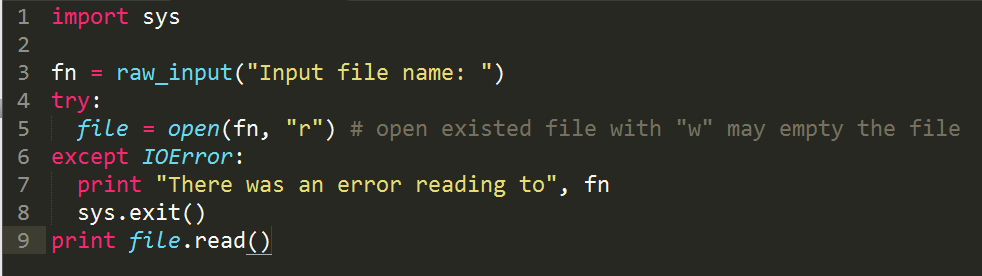 |
Code 3: try-except programming style (i.e. try-catch) |
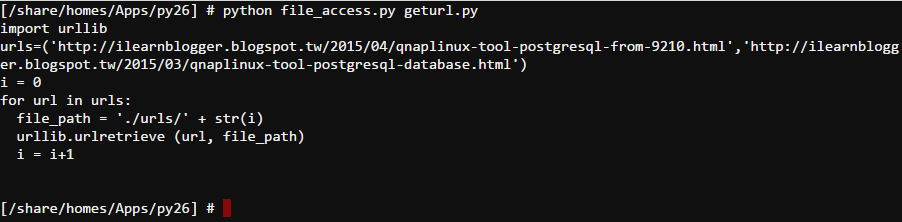 |
Run Code 3 with argument "geturl.py" |
- Multiple Statement Groups as Suits (a block of code statements) after :.
if expression :
suite
elif expression :
suite
else :
suite
Source code:
https://github.com/ilearnblogger/pyp/blob/master/file_access.py
No comments :
Post a Comment